Media Hero
A MediaHero
displays an image or video with optional text, buttons and links positioned as an overlay.
Example
- Preview
- Code
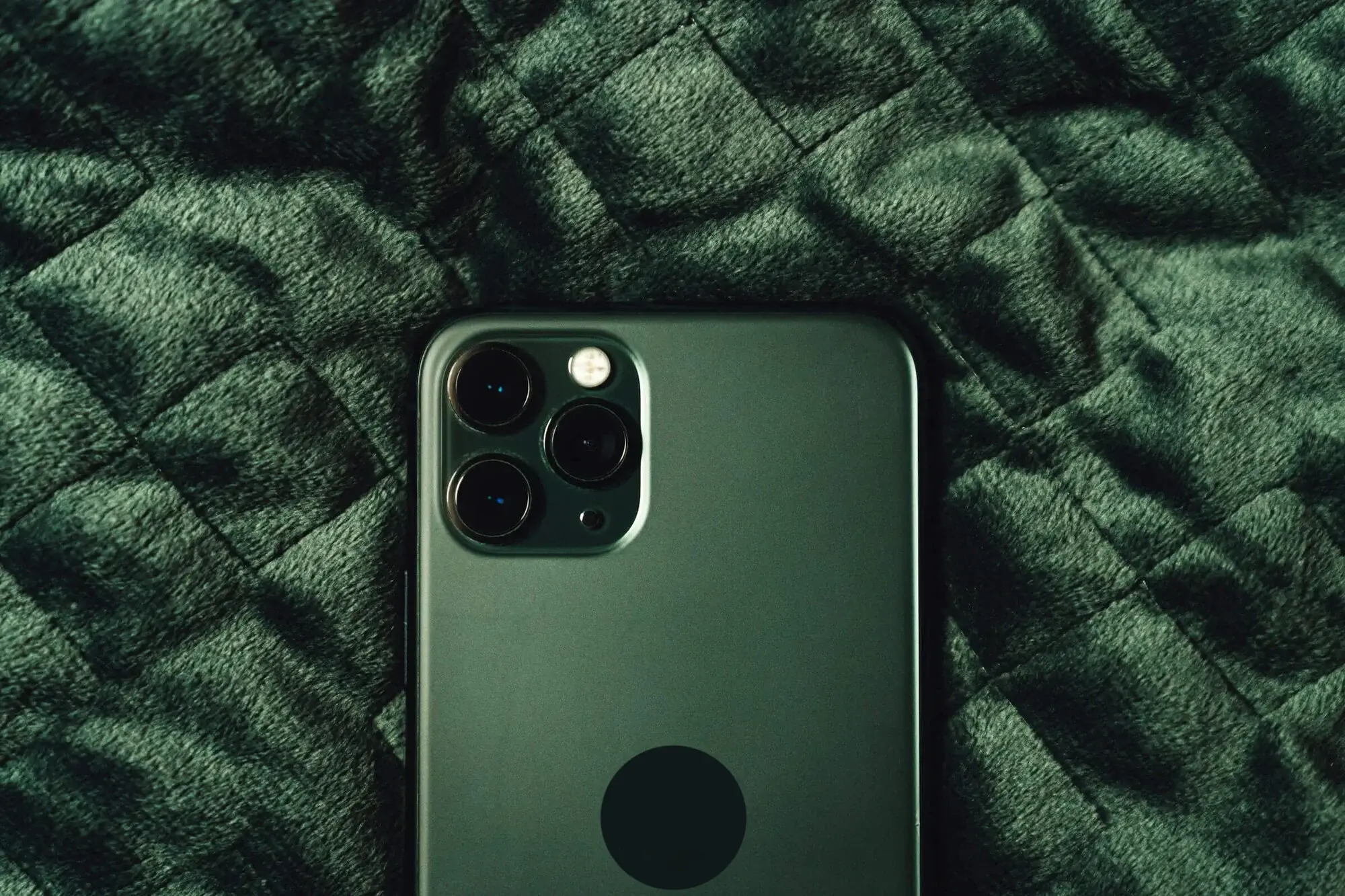
New
Phone
Our most powerful cameras yet. Ultrafast chips. And USB-C.
tip
If using this component in a NextJS app, replace the <img/>
element used in this code example with the NextJS Image
component.
import { Button } from '@hv/ui/button';
import {
MediaHero,
MediaHeroBackground,
MediaHeroCTAs,
MediaHeroContent,
MediaHeroEyebrow,
MediaHeroHeader,
MediaHeroLinks,
MediaHeroSubcopy,
MediaHeroTextBlock,
} from '@hv/ui/hero';
import { ImageLink } from '@hv/ui/image-link';
export default function MediaHeroExample({
textBlockPosition,
ctas,
links,
imageLinks,
media,
eyebrowText,
header,
subcopy,
...variants
}) {
return (
<MediaHero {...variants}>
<MediaHeroBackground>
{media?.type === 'image' && (
<img
src={`/img/${media.src}`}
alt='Mock Media Hero'
className='absolute inset-0 h-full w-full object-cover'
/>
)}
</MediaHeroBackground>
<MediaHeroContent>
<MediaHeroTextBlock position={textBlockPosition}>
<MediaHeroEyebrow {...eyebrowText} />
<MediaHeroHeader {...header} />
<MediaHeroSubcopy {...subcopy} />
</MediaHeroTextBlock>
<MediaHeroCTAs
{...ctas}
direction={variants?.ctaDirection ?? 'rowCenter'}
>
{ctas?.map((cta, idx) => (
<Button key={idx} variant={cta.variant}>
{cta.label}
</Button>
))}
</MediaHeroCTAs>
<MediaHeroLinks
{...links}
direction={variants?.linkDirection ?? 'rowCenter'}
>
{links?.map((link, idx) => (
<Button key={idx} variant='link'>
{link.label}
</Button>
))}
</MediaHeroLinks>
{imageLinks?.length > 0 && (
<MediaHeroLinks
direction={variants?.imageLinkDirection ?? 'rowCenter'}
>
{imageLinks?.map((link, idx) => (
<ImageLink
key={idx}
imageUrl={link.imageUrl ?? ''}
href={link.href ?? ''}
label={link.label}
size={link.size ?? 'button'}
{...link}
/>
))}
</MediaHeroLinks>
)}
</MediaHeroContent>
</MediaHero>
);
}
Variants
Use theicon above to preview
Variant | Description | Values |
---|---|---|
MediaHero.desktopOrientation |
| |
MediaHero.mobileOrientation |
| |
MediaHero.paddingTop |
| |
MediaHero.paddingBottom |
| |
MediaHero.height |
| |
MediaHeroTextBlock.position |
| |
MediaHeroCTAs.direction |
| |
MediaHeroLinks.direction |
| |
MediaHeroLinks.imageLinkDirection |
|
Customization
The approach for customization will vary depending on if the customization is intended to be global for all consumers of the UI component or if it is only an override for a particular instance.
- If the customization is necessary for all users, update the source code in the UI component library directly.
- Otherwise, you can pass
className
overrides.
Say you wanted to make the eyebrow text italic for one particular use case:
<MediaHero>
...
<MediaHeroContent>
<MediaHeroTextBlock>
<MediaHeroEyebrow className='italic'>Eyebrow Text</MediaHeroEyebrow>
<MediaHeroHeader>HeaderText</MediaHeroHeader>
<MediaHeroSubcopy>Subcopy</MediaHeroSubcopy>
</MediaHeroTextBlock>
</MediaHeroContent>
</MediaHero>