Media Card
A MediaCard
displays an image or video with optional text, buttons and links positioned on top.
Example
- Preview
- Code
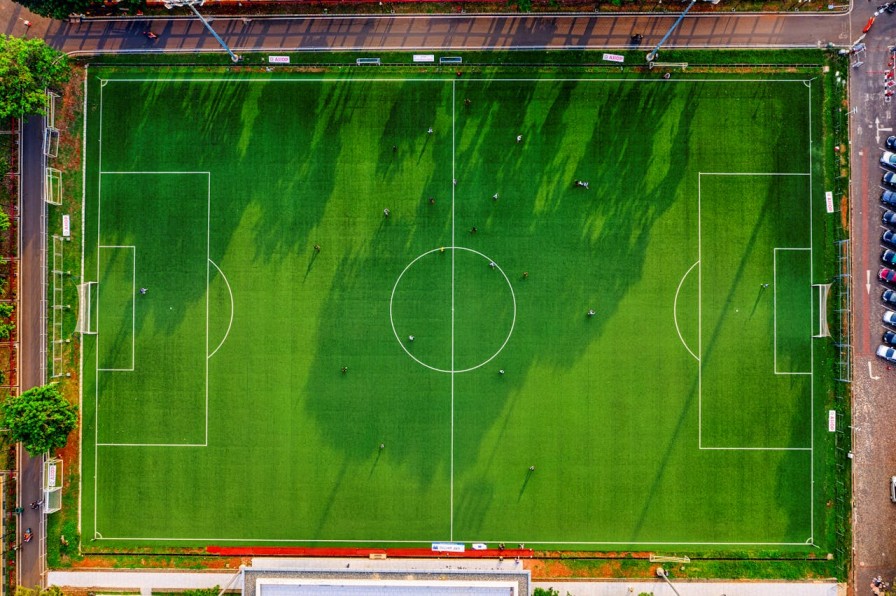
tip
If using this component in a NextJS app, replace the <img/>
element used in this code example with the NextJS Image
component.
import { Button } from '@hv/ui/button';
import {
MediaCard,
MediaCardMedia,
MediaCardBackground,
MediaCardEyebrowText,
MediaCardCTAs,
MediaCardContent,
MediaCardHeader,
MediaCardLinks,
MediaCardSubcopy,
MediaCardTextBlock,
} from '@hv/ui/media-card';
import { ImageLink } from '@hv/ui/image-link';
export default function MediaCardExample({
ctas,
links,
imageLinks,
textBlockPosition,
media,
eyebrowText,
header,
subcopy,
...variants
}) {
return (
<MediaCard
className={
variants.layout === 'stacked'
? 'text-foreground'
: 'text-primary-foreground'
}
{...variants}
>
<MediaCardMedia
layout={variants?.layout ?? 'default'}
height={variants?.height}
>
<MediaCardBackground asChild>
<img
src={`/img/${media.src}`}
alt='Mock Media Card'
className='absolute inset-0 h-full w-full object-cover'
/>
</MediaCardBackground>
</MediaCardMedia>
<MediaCardContent className='bg-muted w-full'>
<MediaCardTextBlock position={textBlockPosition}>
<MediaCardEyebrowText {...eyebrowText} />
<MediaCardHeader {...header} />
<MediaCardSubcopy {...subcopy} />
</MediaCardTextBlock>
<MediaCardCTAs
{...ctas}
direction={variants?.ctaDirection ?? 'rowCenter'}
>
{ctas?.map((cta, idx) => (
<Button asChild key={idx} variant={cta.variant}>
<a href={cta.href ?? ''}>{cta.label}</a>
</Button>
))}
</MediaCardCTAs>
<MediaCardLinks
{...links}
direction={variants?.linkDirection ?? 'rowCenter'}
>
{links?.map((link, idx) => (
<Button asChild key={idx} variant={link.variant}>
<a
href={link.href ?? ''}
className={
variants.layout === 'stacked'
? 'text-foreground hover:text-foreground'
: 'text-primary-foreground hover:text-primary-foreground'
}
>
{link.label}
</a>
</Button>
))}
</MediaCardLinks>
{imageLinks?.length > 0 && (
<MediaCardLinks
direction={variants?.imageLinkDirection ?? 'rowCenter'}
>
{imageLinks?.map((link, idx) => (
<ImageLink
key={idx}
imageUrl={link.imageUrl ?? ''}
href={link.href ?? ''}
label={link.label}
size={link.size ?? 'button'}
{...link}
/>
))}
</MediaCardLinks>
)}
</MediaCardContent>
</MediaCard>
);
}
Variants
Use theicon above to preview
Variant | Description | Values |
---|---|---|
MediaCard.desktopOrientation | Orientation of media card content on desktop viewports |
|
MediaCard.mobileOrientation | Orientation of media card content on mobile viewports |
|
MediaCard.paddingTop | Option to control spacing above the media card |
|
MediaCard.paddingBottom | Option to control spacing below the media card |
|
MediaCard.height | Height control useful for using media card in various contexts |
|
MediaCard.layout | Layout options |
|
MediaCardTextBlock.position | Text alignment of rich text block |
|
MediaCardCTAs.direction | Presentation direction of CTAs |
|
MediaCardLinks.direction | Presentation direction of Links |
|
MediaCardLinks.imageLinkDirection | Presentation direction of Image Links |
|
Customization
The approach for customization will vary depending on if the customization is intended to be global for all consumers of the UI component or if it is only an override for a particular instance.
- If the customization is necessary for all users, update the component source code in the UI library package directly.
- Otherwise, you can pass
className
overrides.
Say you wanted to make the header text larger in one particular instance:
<MediaCard>
...
<MediaCardContent>
<MediaCardTextBlock position={textBlockPosition}>
<MediaCardEyebrowText>{item?.eyebrowText}</MediaCardEyebrowText>
<MediaCardHeader className='text-6xl'>{item?.header}</MediaCardHeader>
<MediaCardSubcopy>{item?.subcopy}</MediaCardSubcopy>
</MediaCardTextBlock>
</MediaCardContent>
</MediaCard>